Web App with HTTP API
CREATE TAG TABLE IF NOT EXISTS EXAMPLE (
NAME VARCHAR(20) PRIMARY KEY,
TIME DATETIME BASETIME,
VALUE DOUBLE SUMMARIZED
);
HTML
Let’s make a html file named simple-webapp.html
. machbase-neo supports .html
, .js
and .css
file editing
Since v8.0.14
.
If you are using previous version of machbase-neo, please update it or use your favorite editor instead.
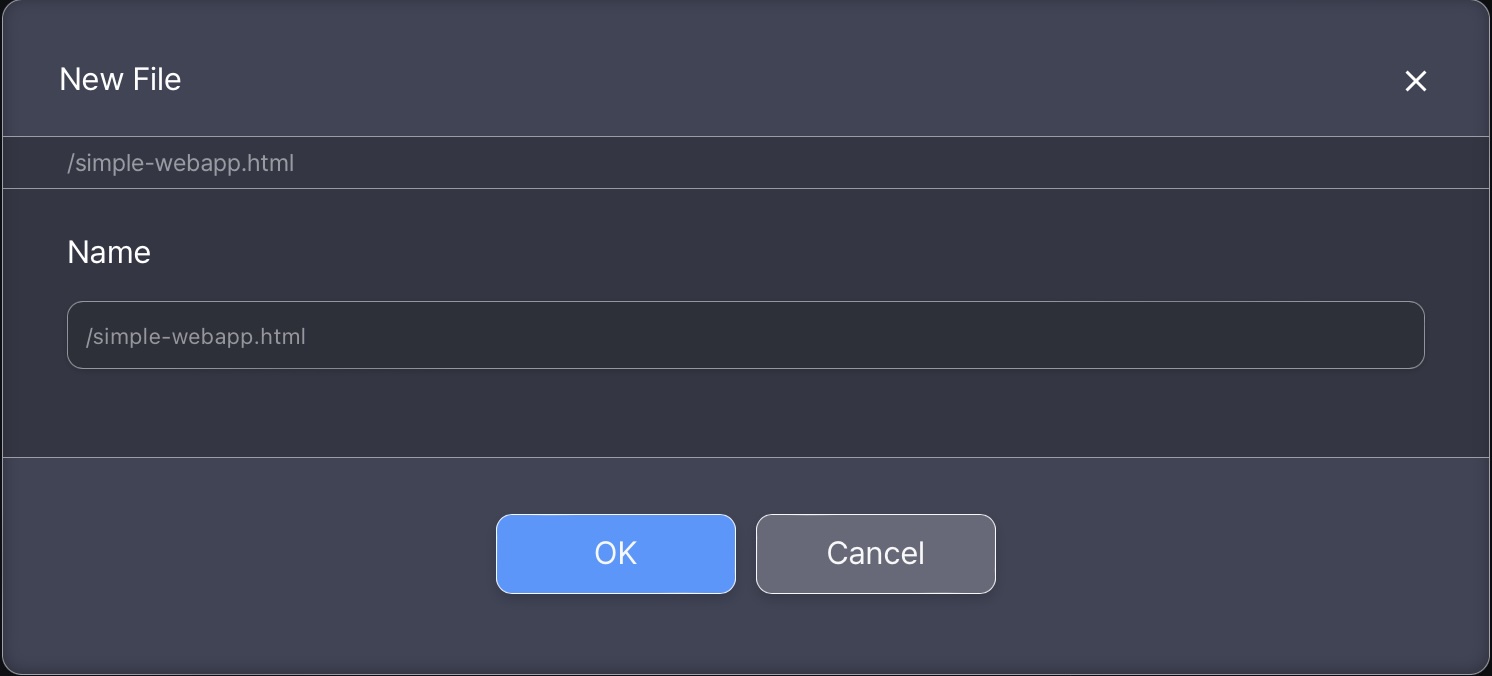
Edit and save html file, open in web browser by click ► button on left top corner of the editor.
Write data
Copy and paste the below codes.
|
|
- line 6:
timeformat=ms
Declare the data payload contains time value in millisecond unix epoch. which is used in line 15Date.now()
. - line 9: Set content-type of the payload, so that machbase-neo interprets it properly.
- line 11-18: The actual data in payload. This example has only one record in the
rows
field, but it may contain multiple records,
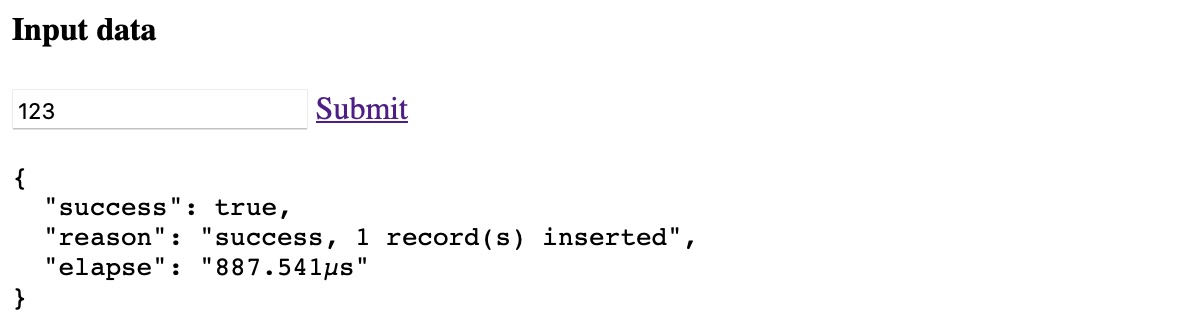
Read data
Extend the simple-webapp.html to read data, add new function queryData(){...}
and “query” action.
|
|
- line 28: Specify the time format of result should be unix epoch in milliseconds.
|
|
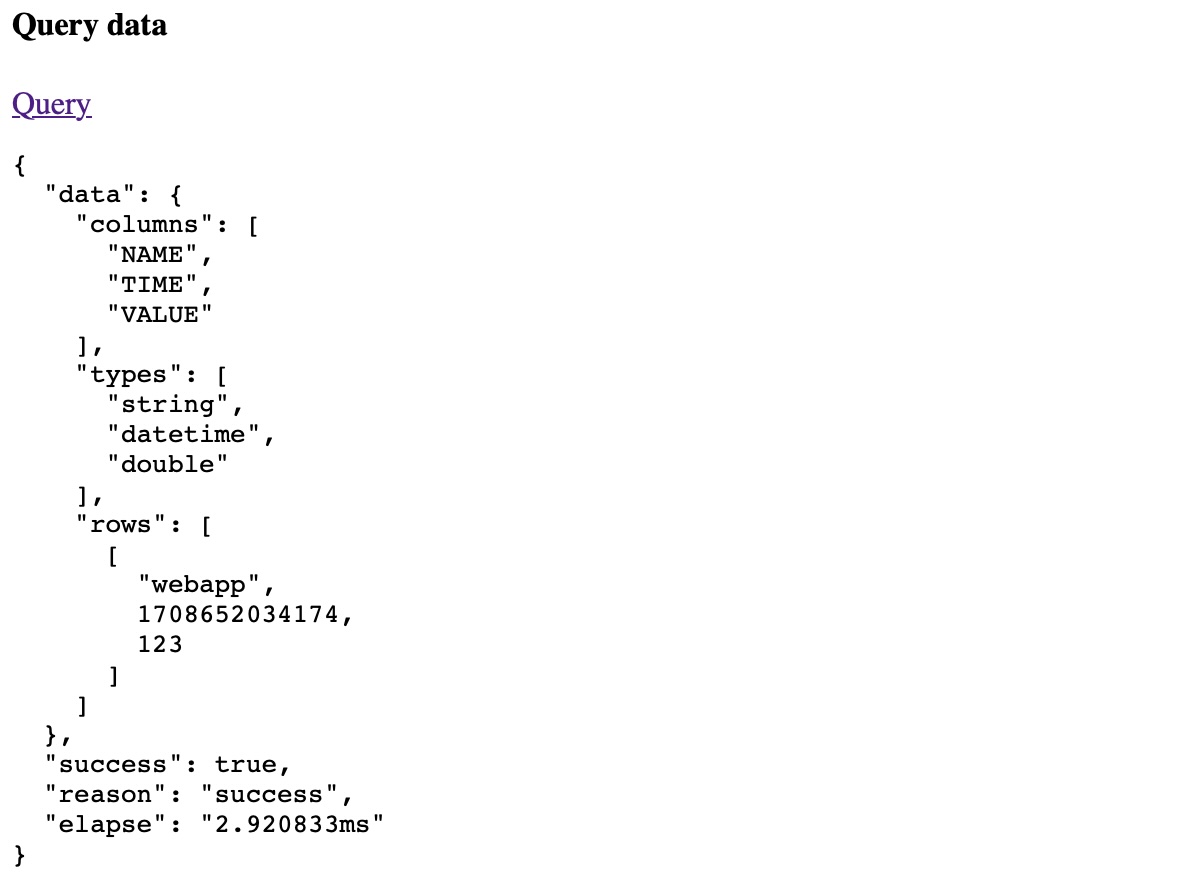
Markdown
The query result can be transform to markdown using TQL, let’s add function markdownData(){...}
and “Markdown” action.
|
|
- line 36: Get output format option if HTML or MARKDOWN text.
- line 40:
MARKDOWN()
TQL SINK with optionshtml(boolean)
,timeformat()
,tz()
…
|
|
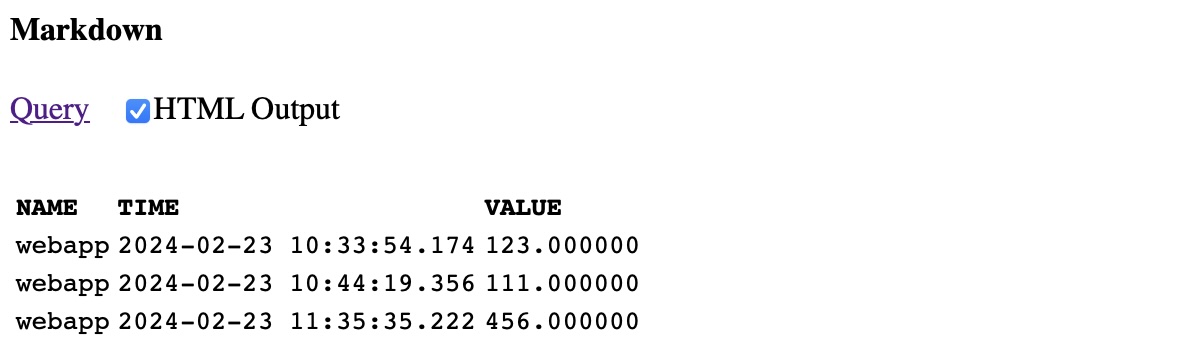
Chart
You can visualize data with any chart library that you prefer by retrieving the query result in JSON and CSV via HTTP API.
But it is still simple and powerful using TQL CHART()
SINK.
This example shows how to visualize data with TQL without any extra tool.
Add function chartData()
and a helper function loadJS()
.
|
|
And add Apache echart library, it is already included in machbase-neo for pre-loading.
|
|
|
|
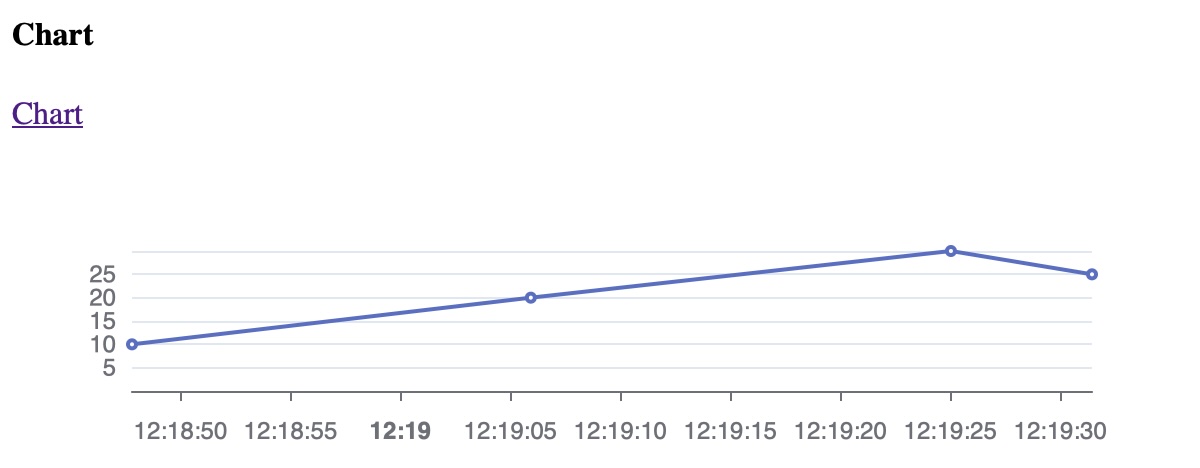
Full source code
|
|